Tasks studies - laboratory
To load files, we can use the StreamReader class, which allows us to open, read and close a text file. We pass the file path through the StreamReader constructor to automatically open the file. The ReadLine method reads each line of text and increments the file pointer to the next line as it reads. When the ReadLine method reaches the end of the file, it returns a null reference.
//We open the stream file sample.txt
using (var sr = new StreamReader("sample.txt"))
{
var line = sr.ReadLine();
while (line != null)
{
Console.WriteLine(line);
//Load the next line
line = sr.ReadLine();
}
}
We can also use the ReadToEnd method, which reads the entire stream to the end from the current position.
//We open the stream of the sample.txt file
using (var sr = new StreamReader("sample.txt"))
{
var line = sr.ReadToEnd();
Console.WriteLine(line);
}
To write data to a file, I will use the StreamWriter class, which allows you to open, save, and close a text file. In a similar way to the StreamReader class, we pass the file path to the StreamWriter constructor to automatically open the file. The WriteLine method writes a full line of text to a text file. ``` //We open the stream of the file sample.txt using (var sw = new StreamWriter(“sample.txt”)) { sw.WriteLine(“Hello World!!”); sw.WriteLine(“From the StreamWriter class”); }
To append values to the file (and not overwrite it), we need to set the append flag to true in the StreamWriter constructor.
//We open the stream of the file sample.txt using (var sw = new StreamWriter(“sample.txt”, append: true)) { sw.WriteLine(“Hello World!!”); sw.WriteLine(“From the StreamWriter class”); }
### JSON
JSON (JavaScript Object Notation) is a simple data exchange format. Writing and reading data in
this format is easy for humans to learn. At the same time, it is easy for computers to read and generate. Its definition is based on a subset of the JavaScript programming language. JSON is a text format
that is completely independent of programming languages, but uses conventions that are familiar
to programmers using languages in the C family, including C++, C#, Java, JavaScript, Perl, Python, and
many others. These properties make JSON an ideal language for data exchange. JSON is based
on two structures:
· A set of name/value pairs. In various languages, this is implemented as an object, record,
structure, dictionary, hash table, keyed list, or associative table.
· An ordered list of values. In most languages, this is implemented using a table,
vector, list, or sequence.
In the case of JSON, these take the following forms:
An object is an unordered set of name/value pairs. The object description starts with a {left brace and ends with a }right brace. Each name is followed by a :colon and name/value pairs, separated by a comma.
<br>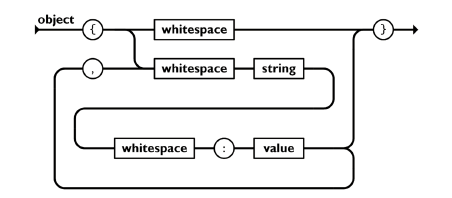
These data structures are universal. Almost all modern programming languages
use them in one form or another. It makes sense that a data format that is portable between
different programming languages should base its structure on these structures.
{ “fruit”: “Apple”, “size”: “Large”, “color”: “Red” }
### Serialization of a JSON file
To make serialization and deserialization of a JSON file easier, we will use the Newtonsoft.Json library.
### Deserialization:
JsonConvert.DeserializeObject
### Tasks to do yourself:
### 1.
Write a program that allows you to save the album number of the person who
wrote the program to a file with a specified name.
### 2.
Write a program that allows you to load the contents of a file with a specified name.
### 3.
Write a program that loads a list of PESELs from the file pesels.txt, and then implement a function
that checks how many female PESELs there are in the loaded set. ### 4.
Using the `db.json` database, containing information about the population of the USA, India, and China since 1960, write a program:
· Allows you to check the population difference between 1970 and 2000 for India
· Allows you to check the population difference between 1965 and 2010 for the USA
· Allows you to check the population difference between 1980 and 2018 for China
· Allows the user to select the year and country from which they would like to display the population.
· Allows the user to check the population difference for a given range of years and
countries,
· Allows the user to check the percentage population growth for each
country relative to the previous year to the specified year,
### 5.
Write a program that implements the IPersonRepository interface, via the FilePersonRepository class
working on a file database. (Json, record per line, etc.).